How to use JSON in Angular
In this article, I’ll explain how to use JSON in an Angular web application using two different approaches. Let’s start.
Setup the Angular application
Create a new Angular application:
ng new test-app
and
cd test-app
Open the project in your favorite code editor and navigate to src/app/app.component.html
and replace everything with just the following line of code:
<h1>Using JSON in Angular</h1>
Run your local server with ng serve
and navigate to http://localhost:4200, you should see something like this:
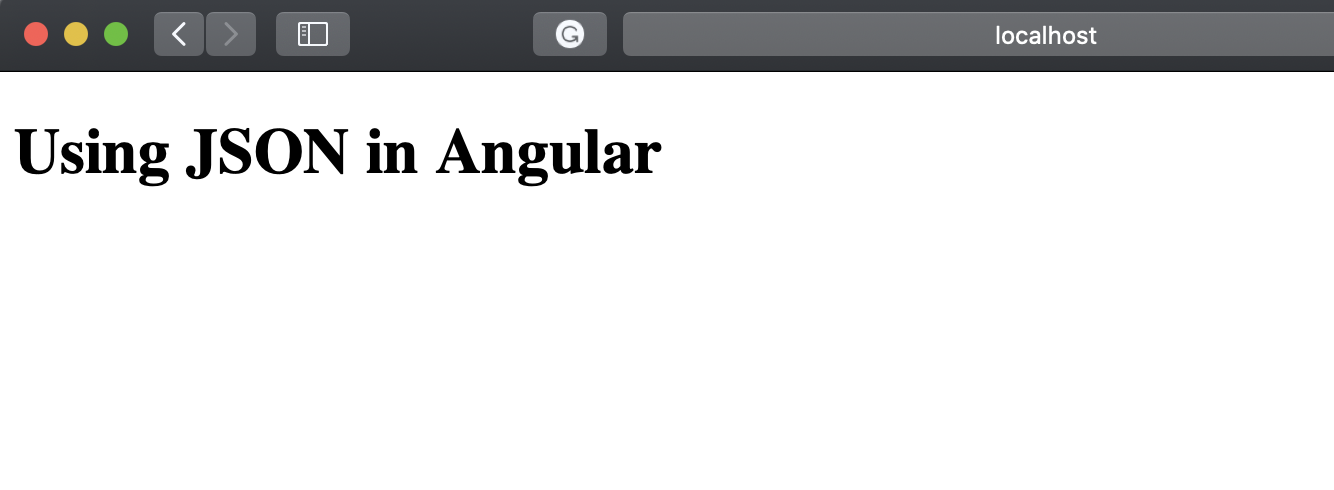
Now, with our app all set up, let’s explore the two different methods to use JSON in Angular.
Method #1: JSON in Angular with a TypeScript Module
Since Typescript 2.9, we can import JSON files as regular Typescript modules by simply enabling it in our tsconfig.json file.
Open tsconfig.spec.json
and add “resolveJsonModule”: true, as shown below:
/* To learn more about this file see: https://angular.io/config/tsconfig. */
{
"extends": "./tsconfig.base.json",
"compilerOptions": {
"outDir": "./out-tsc/spec",
"resolveJsonModule": true,
"types": [
"jasmine"
]
},
"files": [
"src/test.ts",
"src/polyfills.ts"
],
"include": [
"src/**/*.spec.ts",
"src/**/*.d.ts"
]
}
src/app/
folder and paste the following product JSON data:
[
{
"id": 1,
"name": "Elegance Hair Gel",
"description": "Strong hold hair gel.",
"price": 9.99,
"stock": 10567
},
{
"id": 2,
"name": "Elegance Hair Serum",
"description": "Hair serum with vitamins.",
"price": 14.99,
"stock": 9956
},
{
"id": 3,
"name": "Elegance Hair Shampoo",
"description": "Hair Shampoo with Aloe Vera.",
"price": 7.99,
"stock": 5689
}
]
Next, we need to import the JSON data to our app component by adding import * as data from ‘./sample.json’; in the top import section in our app.component.ts
file.
Now the app component has access to our JSON file but we still need to read the data. Let’s define and assign the JSON data to a variable in our class component: products: any = (data as any).default;.
Your app.component.ts file should look similar to this:
import { Component } from '@angular/core';
import * as data from './sample.json';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'test-app';
products: any = (data as any).default;
}
Finally, we need to display our data in our template. Open up app.component.html
and add the following unordered list:
<h1>Using JSON in Angular</h1>
<ul>
<li *ngFor="let product of products">
{{product.name}}
</li>
</ul>
Here, we are just iterating through the name
values of our product
data with *ngFor
and displaying each value in a list.
Run your server and go to http://localhost:4200. You should see something like this:
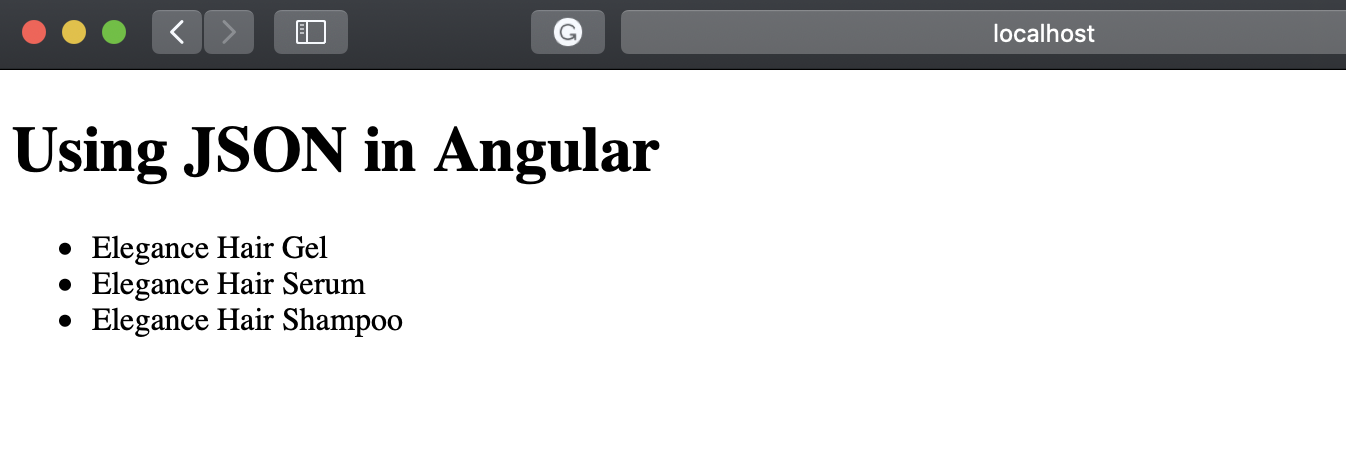
Method #2: JSON in Angular with an HTTP Request
If you are trying to use JSON through an HTPP request with your web application, Angular provides a client HTTP API, which provides some excellent features.
To use it, we first need to import the HTTPClientModule in our app. Open app/app.module.ts
and add two lines of code, as shown below.
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { HttpClientModule } from '@angular/common/http';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Next, open app.component.ts
, and let’s add some code. First, we need to import the HTPPClient module at the top:
import { HttpClient } from '@angular/common/http';
After importing the HTTPclient module in our component, we can either use a local JSON file or a REST API. We’ll cover both next.
Using a REST API
We will be using a free service called JSONPlaceholder to retrieve JSON data and test our code. We can create a function to make a GET request to retrieve users data by adding the following code:
constructor(public httpClient: HttpClient){}
giveMeData(){
this.httpClient.get('https://jsonplaceholder.typicode.com/users').subscribe((resp)=>{
this.var1 = resp;
});
}
Your final app.component.ts
file should look like this:
import { Component } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'test-app';
constructor(public httpClient: HttpClient){}
giveMeData(){
this.httpClient.get('https://jsonplaceholder.typicode.com/users').subscribe((resp)=>{
this.var1 = resp;
});
}
}
Finally, let’s display our data in our app.component.html
template by adding a button to retrieve the names in a list.
<h1>Using JSON in Angular</h1>
<button (click)="giveMeData()">GET Users</button>
<ul>
<li *ngFor="let nombre of var1">
{{nombre.name}}
</li>
</ul>
Run your server with ng serve and you should get:
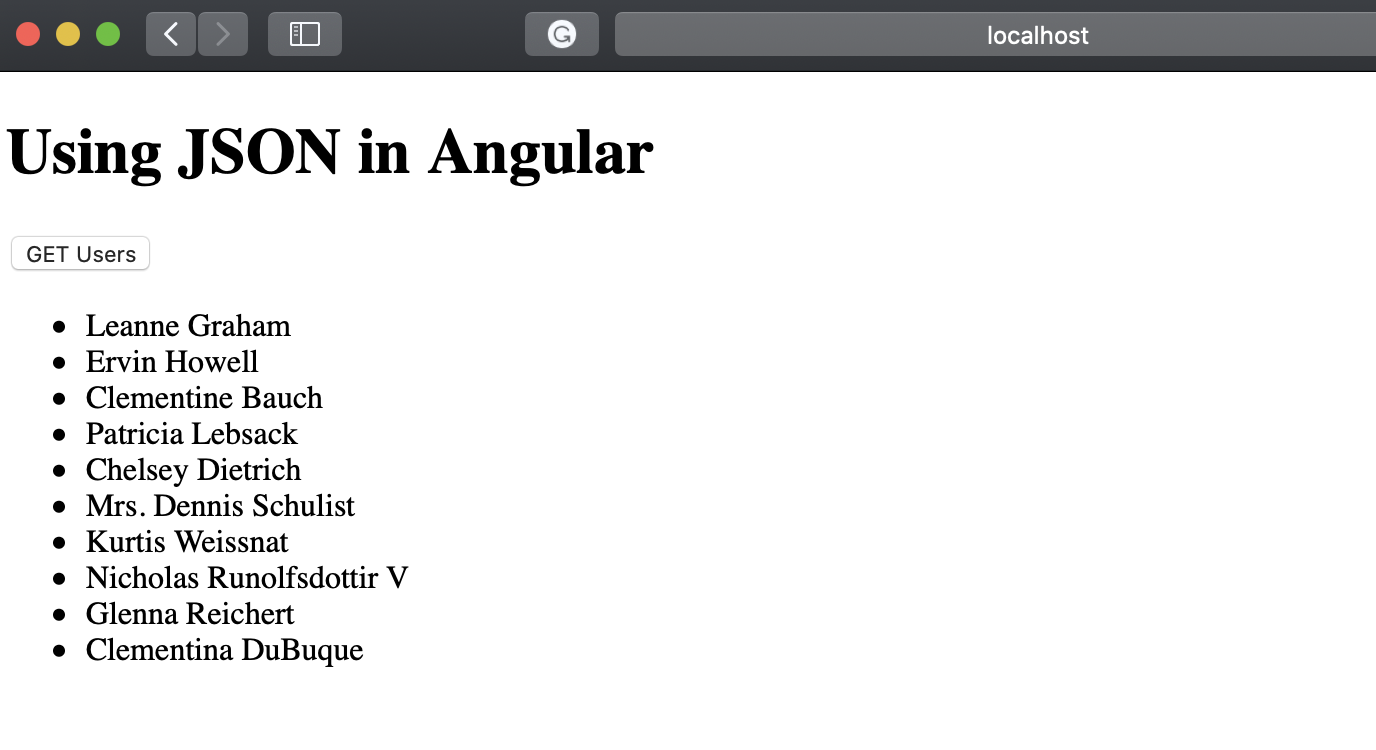
Using a local JSON file
We can also use a local JSON file by uploading it to our src/assets/
folder and modify our giveMeData()
function as follows:
import { Component } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'test-app';
constructor(public httpClient: HttpClient){}
giveMeData(){
this.httpClient.get('assets/sample.json').subscribe((resp)=>{
this.var1 = resp;
});
}
}
And there you have it! A couple of methods to use JSON in an Angular web app. Happy coding!