If you find yourself using the same code or content across your web pages, or need to add some functionality, it might be a good time to start using WordPress shortcodes.
What are WordPress shortcodes?
WordPress shortcodes are pieces of code added to your theme files that dynamically add content into pages, posts, and widgets.
You can call the shortcode by merely adding the shortcode’s name inside square brackets like this:
[sampleshortcode]
The image below illustrates how to insert a shortcode with the name banner into a WordPress page using the code editor.
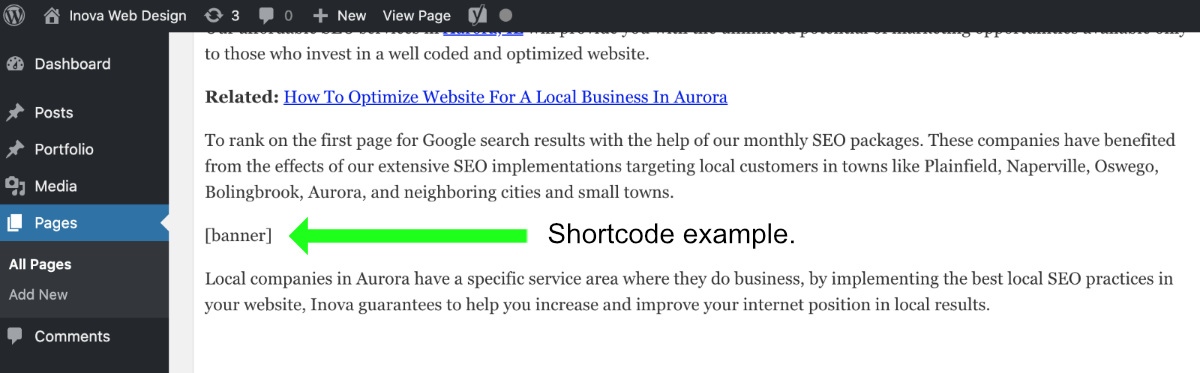
Why use a shortcode instead of just code?
You can write text, HTML, Javascript, and other content in the WordPress page/post editor, but you can not add PHP code. If you need to add a particular functionality, reuse code, add a custom banner, or gallery, then a shortcode can help you with that.
How to create a WordPress shortcode
You can create a shortcut by adding the code you need inside a PHP function and registering it as a shortcode. Once WordPress detects a shortcode, it calls the function and displays the code inside of it.
Let’s create some shortcodes to demonstrate how they work.
Example #1
Basic Hello World! WordPress shortcode example
First, we need to add the shortcode function inside one of the theme files. You can create a new file or create the function inside one of the other theme files.
Where to place the shortcode function?
Placing shortcodes inside the functions.php file or the /inc/template-functions.php file is a good common practice.
For our example, we will create a new file called my-shortcode-functions.php inside the inc (includes) folder.
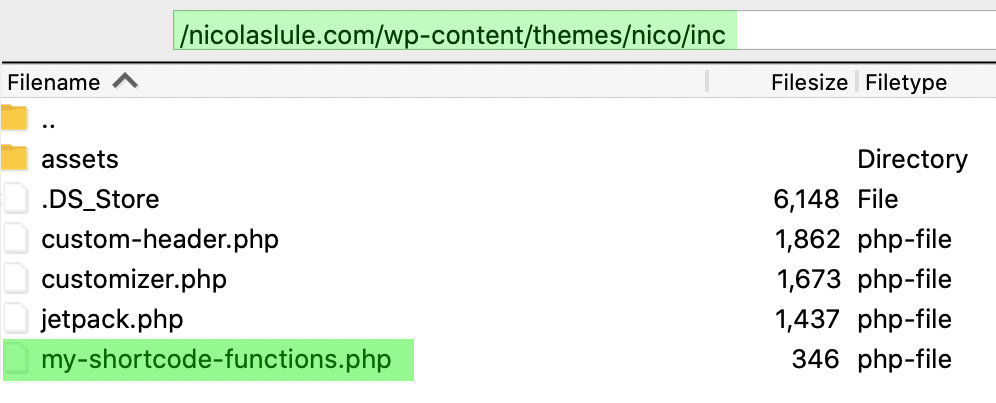
Next, let’s add our shortcode with a PHP function to the /inc/template-functions.php file:
<?php
// First, we declare the function
function my_custom_shortcode() {
// Code to run. We store a string message in the $msg variable.
$msg = 'Hello world!';
// We return the $msg text to be displayed.
return $msg;
}
// Finally, we register the shortcode with our custon name "abcdef".
add_shortcode('abcdef', 'my_custom_shortcode');
First, we declare the function with the name my_custom_shortcode
that runs some very basic code and returns the “Hello world!” string stored in the $msg
variable. Finally, we register the shortcode with the WordPress add_shortcode
function.
Now you can use the shortcode on your posts, pages, and widgets by simply adding it in the WordPress code editor:
[abcdef]
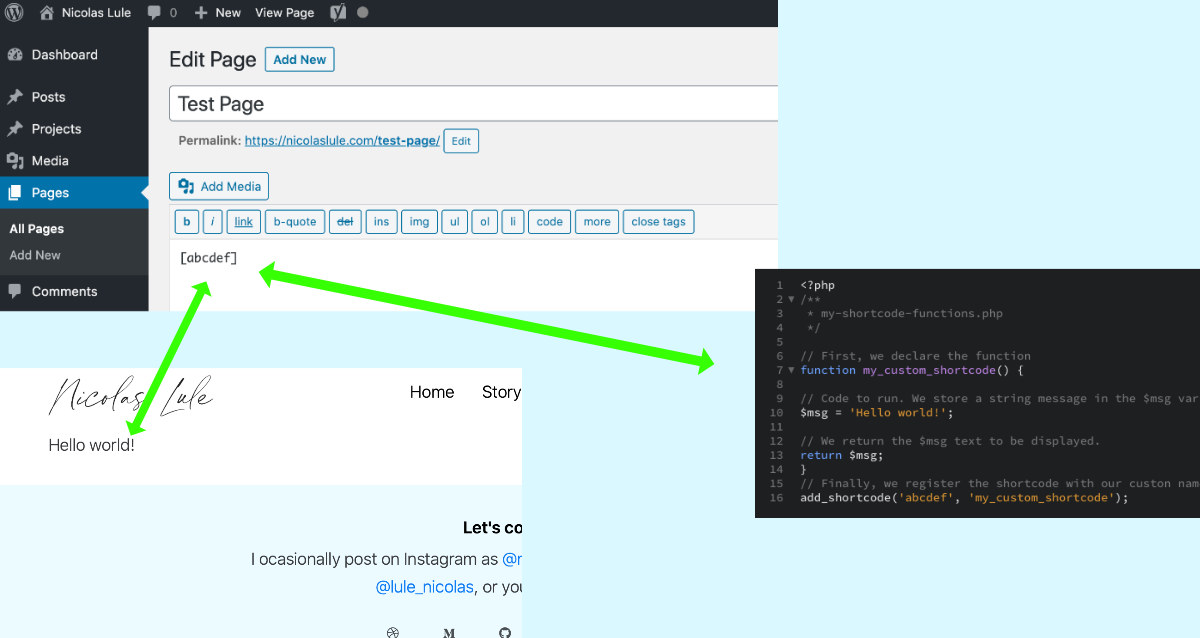
Learn more about the add_shortcode function in the official WordPress documentation.
Example #2
HTML WordPress shortcode example
Shortcodes are not limited to returning just strings. You can also return HTML code as shown in the following example.
<?php
/** my-shortcode-functions.php*/
// First, we declare the function
function my_custom_shortcode() {
// Code to run. We store the HTML code in the $msg variable.
$msg = '<h1>Hello world!</h1>';
// We return the $msg variable containing the HTML code.
return $msg;
}
// Finally, we register the shortcode with our custon name "abcdef".
add_shortcode('abcdef', 'my_custom_shortcode');
Example #3
WordPress shortcode with attributes example
HTML + dynamic content shortcode
Shortcodes are flexible and allow us to use parameters (attributes) to customize and add content dynamically. Think of parameters as data you pass to customize the result of the shortcode.
In order to use parameters, we need to declare them using the WordPress’ built-in shortcode_atts() function as shown in the following example.
<?php
function my_custom_shortcode( $atts ) {
$a = shortcode_atts( array(
'hello-term' => 'world!',
), $atts);
ob_start(); ?>
<h1>Hello <?php echo $a['hello-term'] ?></h1>
<?php
$code_var = ob_get_clean();
return $code_var;
}
add_shortcode( 'abcdef', 'my_custom_shortcode' );
We first declare the attributes with the $atts
variable. We store the array of parameters in the $a
variable as key-value pairs. We then use the hello-term attribute in the shortcode content by using the PHP echo
function.
We use PHP output buffering to be able to display our attributes inside the HTML code. Finally, we return the complete HTML code stored in the $code_var
.
We use the shortcode in the WordPress editor as follows:
[abcdef hello-term='galaxy!']
And this should be displayed on the webpage as:
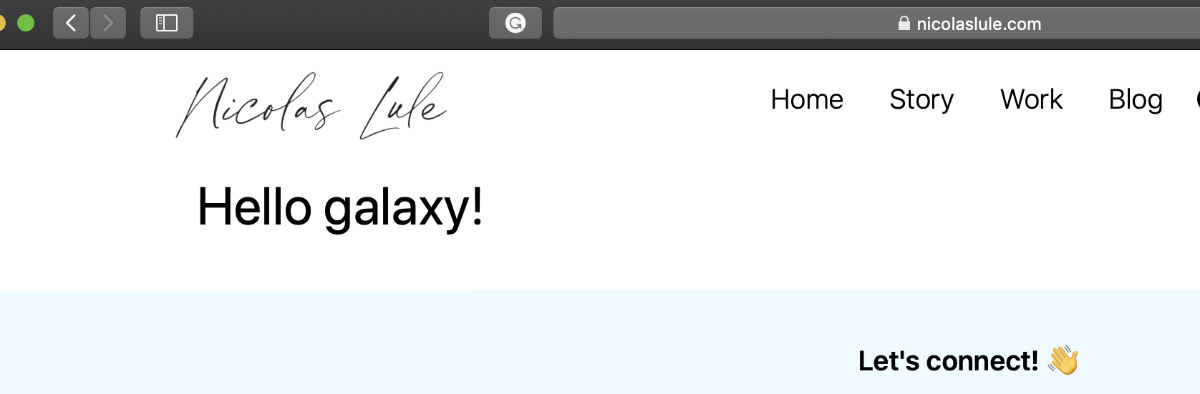
Note:
The default hello-term value is world! as expressed by this line of code in the function
'hello-term' => 'world!'
You can learn more about PHP output buffering here. Now that we understand how shortcodes work and how to use them, we will use them for more practical situations.
Example #4
Structured data (schema) WordPress shortcode example
Implementing a shortcode makes sense when you have to repeat a lot of code in different webpages.
Take, for example, a Chicago nightclub website that posts weekly events and needs to add the Event Schema to attract more organic site visitors and display rich snippets in Google searches.
<?php
function schema_play_event($schema_event_atts) {
$schema = shortcode_atts(
array(
'event-name' => '',
'event-image' => '',
'event-desc' => '',
'event-url' => '',
'event-start' => '',
'event-end' => '',
'performer-image' => '',
'performer-name' => '',
'performer-link' => ''
), $schema_event_atts);
ob_start(); ?>
<script type="application/ld+json">// <![CDATA[
{
"@context" : "http://schema.org",
"@type" : "Event",
"name" : "<?php echo htmlentities($schema['event-name'], ENT_QUOTES); ?>",
"image": "<?php echo htmlentities($schema['event-image'], ENT_QUOTES); ?>",
"description": "<?php echo htmlentities($schema['event-desc'], ENT_QUOTES); ?>",
"url": "<?php echo htmlentities($schema['event-url'], ENT_QUOTES); ?>",
"startDate" : "<?php echo htmlentities($schema['event-start'], ENT_QUOTES); ?>",
"endDate": "<?php echo htmlentities($schema['event-end'], ENT_QUOTES); ?>",
"performer": [
{
"@type": "Person",
"image": "<?php echo htmlentities($schema['performer-image'], ENT_QUOTES); ?>",
"name": "<?php echo htmlentities($schema['performer-name'], ENT_QUOTES); ?>",
"sameAs": "<?php echo htmlentities($schema['performer-link'], ENT_QUOTES); ?>"
}
],
"location" : {
"@type" : "Place",
"name" : "Play Kitchen & Cocktails",
"address" : {
"@type" : "PostalAddress",
"streetAddress" : "7 W Division St",
"addressLocality" : "Chicago",
"addressRegion" : "IL",
"postalCode" : "60610" }
},
"typicalAgeRange" : "18+"
}
// ]]>
</script>
<?php
$schema_content = ob_get_clean();
return $schema_content;
}
add_shortcode( 'play-event', 'schema_play_event' );
As you can see, they just need to add the shortcode in the post for the event to add JSON-LD structured data as follows:
[play-event event-name='Live Music DJ Kid Clay' event-image='https://theplaychicago.com/wp-content/uploads/D11AEDD2-7398-42DD-9D48-DBADE51CA65C.jpeg' event-desc='Join us Friday, October 4th for a fun night with DJ Kid Clay and enjoy delicious cocktails, wine, beer, and food.' event-url='https://theplaychicago.com/friday-october-4th-with-heavy/' event-start='2019-10-04T16:00' event-end='2019-10-04T04:00' performer-image='http://kidclay.com/wp-content/uploads/2018/02/kc1232-3.png' performer-name='Kid Clay' performer-link='http://kidclay.com/']